This is not a guide to JavaScript callbacks, promise, async/await works, there are many free tutorials made by professionals and simple to find on google
Prerequisites:
NodeJs 7.6+
npm mysql
For this example, I’ll use the query SELECT sleep(x) to return 0 after x seconds
Let’s see the code:
pool.query('SELECT sleep(6)', (err, results) => {
if (err) console.log(err);
console.log('End query 1 (6 seconds)');
});
pool.query('SELECT sleep(3)', (err, results) => {
if (err) console.log(err);
console.log('End query 2 (3 seconds)');
});
The result is:

The two queries were run in parallel and the second one finished first.
This is very powerful, but in some cases, we may need to wait until the end of the first query before running the second one.
const esecuzione = async function() {
try {
await new Promise((res, rej) => {
pool.query('SELECT sleep(5)', (err, results) => {
if (err) return rej(err);
res(results);
console.log('End query 1 (5 seconds)');
});
});
await new Promise((res, rej) => {
pool.query('SELECT sleep(3)',(err, results) => {
if (err) return rej(err);
res(results);
console.log('End query 2 (3 seconds)');
});
});
} catch (err) {
console.log(err)
}
}
The result is:
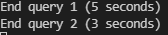
The two queries was fired sequentially inside an async function
How does it work?
First I “promisified” the pool.queries : await new Promise((res, rej) => {
Then I put those promises inside a try/catch statement to intercept the error.
res <- resolved
rej <- Rejected
The second query is (await) awaiting the resolution of the first query res(results);
If there is an error in the query, the promise gets rejected if (err) return rej(err); then the error is caught by the catch statement and printed avoiding the server crash.
Questions? Suggestions? Please leave a comment below.